图书介绍
C++ how to programPDF|Epub|txt|kindle电子书版本网盘下载
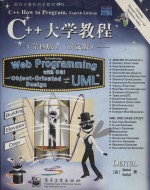
- Fourth Edition = C++大学教程(第四版)(英文版) 著
- 出版社: Publishing House of Electronics Industry
- ISBN:7121017598
- 出版时间:2005
- 标注页数:1342页
- 文件大小:194MB
- 文件页数:1407页
- 主题词:C语言-程序设计-高等学校-教材-英文
PDF下载
下载说明
C++ how to programPDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
1 Introduction to Computers and C++ Programming1
1.1 Introduction2
1.2 What is a Computer?4
1.3 Computer Organization5
1.4 Evolution of Operating Systems6
1.5 Personal Computing,Distributed Computing and Client/Server Computing7
1.6 Machine Languages,Assembly Languages,and High-level Languages7
1.7 History of C and C++8
1.8 C++ Standard Library10
1.9 Java11
1.10 Visual Basic,Visual C++ and C11
1.11 Other High-level Languages13
1.12 Structured Programming13
1.13 The Key Software Trend:Object Technology14
1.14 Basics of a Typical C++ Environment15
1.15 Hardware Trends17
1.16 History of the Internet18
1.17 History of the World Wide Web19
1.18 World Wide Web Consortium (W3C)20
1.19 General Notes About C++ and This Book20
1.20 Introduction to C++ Programming21
1.21 A Simple Program:Printing a Line of Text21
1.22 Another Simple Program:Adding Two Integers26
1.23 Memory Concepts30
1.24 Arithmetic31
1.25 Decision Making:Equality and Relational Operators34
1.26 Thinking About Objects:Introduction to Object Technology and the Unified Modeling Language40
1.27 Tour of the Book44
2 Control Structures70
2.1 Introduction71
2.2 Algorithms72
2.3 Pseudocode72
2.4 Control Structures73
2.5 if Selection Structure76
2.6 if/else Selection Structure77
2.7 while Repetition Structure81
2.8 Formulating Algorithms:Case Study 1 (Counter-Controlled Repetition)83
2.9 Formulating Algorithms with Top-Down,Stepwise Refinement:Case Study 2 (Sentinel-Controlled Repetition)86
2.10 Formulating Algorithms with Top-Down,Stepwise Refinement:Case Study 3 (Nested Control Structures)94
2.11 Assignment Operators98
2.12 Increment and Decrement Operators99
2.13 Essentials of Counter-Controlled Repetition102
2.14 for Repetition Structure104
2.15 Examples Using the for Structure109
2.16 switch Multiple-Selection Structure113
2.17 do/while Repetition Structure120
2.18 break and continue Statements122
2.19 Logical Operators124
2.20 Confusing Equality (==) and Assignment (=) Operators127
2.21 Structured-Programming Summary128
2.22 [Optional Case Study] Thinking About Objects:Identifying a System’s Classes from a Problem Statement133
3 Functions169
3.1 Introduction170
3.2 Program Components in C++170
3.3 Math Library Functions171
3.4 Functions173
3.5 Function Definitions174
3.6 Function Prototypes178
3.7 Header Files180
3.8 Random Number Generation182
3.9 Example:Game of Chance and Introducing enum188
3.10 Storage Classes192
3.11 Scope Rules195
3.12 Recursion198
3.13 Example Using Recursion:Fibonacci Series202
3.14 Recursion vs.Iteration206
3.15 Functions with Empty Parameter Lists208
3.16 Inline Functions209
3.17 References and Reference Parameters211
3.18 Default Arguments215
3.19 Unary Scope Resolution Operator217
3.20 Function Overloading219
3.21 Function Templates222
3.22 [Optional Case Study] Thinking About Objects:Identifying a Class’s Attributes225
4 Arrays252
4.1 Introduction253
4.2 Arrays253
4.3 Declaring Arrays255
4.4 Examples Using Arrays256
4.5 Passing Arrays to Functions272
4.6 Sorting Arrays276
4.7 Case Study:Computing Mean,Median and Mode Using Arrays278
4.8 Searching Arrays:Linear Search and Binary Search283
4.9 Multiple-Subscripted Arrays289
4.10 [Optional Case Study] Thinking About Objects:Identifying the Operations of a Class296
5 Pointers and Strings319
5.1 Introduction320
5.2 Pointer Variable Declarations and Initialization320
5.3 Pointer Operators322
5.4 Calling Functions by Reference325
5.5 Using const with Pointers329
5.6 Bubble Sort Using Pass-by-Reference336
5.7 Pointer Expressions and Pointer Arithmetic341
5.8 Relationship Between Pointers and Arrays344
5.9 Arrays of Pointers349
5.10 Case Study:Card Shuffling and Dealing Simulation350
5.11 Function Pointers355
5.12 Introduction to Character and String Processing360
5.12.1 Fundamentals of Characters and Strings360
5.12.2 String Manipulation Functions of the String-Handling Library362
5.13 [Optional Case Study] Thinking About Objects:Collaborations Among Objects370
6 Classes and Data Abstraction404
6.1 Introduction405
6.2 Structure Definitions406
6.3 Accessing Structure Members407
6.4 Implementing User-Defined Type Time with a C-like struct408
6.5 Implementing Abstract Data Type Time with a class411
6.6 Class Scope and Accessing Class Members418
6.7 Separating Interface from Implementation420
6.8 Controlling Access to Members424
6.9 Access Functions and Utility Functions426
6.10 Initializing Class Objects:Constructors430
6.11 Using Default Arguments with Constructors430
6.12 Destructors435
6.13 When Constructors and Destructors Are Called435
6.14 Using Set and Get Functions439
6.15 Subtle Trap:Returning a Reference to a private Data Member445
6.16 Default Memberwise Assignment448
6.17 Software Reusability450
6.18 [Optional Case Study] Thinking About Objects:Starting to Program the Classes for the Elevator Simulator451
7 Classes:Part Ⅱ468
7.1 Introduction469
7.2 const (Constant) Objects and const Member Functions469
7.3 Composition:Objects as Members of Classes478
7.4 friend Functions and friend Classes485
7.5 Using the this Pointer489
7.6 Dynamic Memory Management with Operators new and delete495
7.7 static Class Members497
7.8 Data Abstraction and Information Hiding502
7.8.1 Example:Array Abstract Data Type504
7.8.2 Example:String Abstract Data Type504
7.8.3 Example:Queue Abstract Data Type505
7.9 Container Classes and Iterators505
7.10 Proxy Classes506
7.11 [Optional Case Study] Thinking About Objects:Programming the Classes for the Elevator Simulator509
8 Operator Overloading; String and Array Objects546
8.1 Introduction547
8.2 Fundamentals of Operator Overloading548
8.3 Restrictions on Operator Overloading549
8.4 Operator Functions as Class Members vs.as friend Functions550
8.5 Overloading Stream-Insertion and Stream-Extraction Operators552
8.6 Overloading Unary Operators555
8.7 Overloading Binary Operators555
8.8 Case Study:Array Class556
8.9 Converting between Types568
8.10 Case Study:string Class569
8.11 Overloading ++ and --581
8.12 Case Study:A Date Class582
8.13 Standard Library Classes string and vector588
9 Object-Oriented Programming:Inheritance609
9.1 Introduction610
9.2 Base Classes and Derived Classes611
9.3 protected Members614
9.4 Relationship between Base Classes and Derived Classes614
9.5 Case Study:Three-Level Inheritance Hierarchy637
9.6 Constructors and Destructors in Derived Classes642
9.7 “Uses A” and “Knows A” Relationships648
9.8 public,protected and private Inheritance648
9.9 Software Engineering with Inheritance649
9.10 [Optional Case Study] Thinking About Objects:Incorporating Inheritance into the Elevator Simulation650
10 Object-Oriented Programming:Polymorphism662
10.1 Introduction663
10.2 Relationships Among Objects in an Inheritance Hierarchy664
10.2.1 Invoking Base-Class Functions from Derived-Class Objects665
10.2.2 Aiming Derived-Class Pointers at Base-Class Objects670
10.2.3 Derived-Class Member-Function Calls via Base-Class Pointers672
10.2.4 Virtual Functions673
10.3 Polymorphism Examples679
10.4 Type Fields and switch Structures680
10.5 Abstract Classes680
10.6 Case Study:Inheriting Interface and Implementation682
10.7 Polymorphism,Virtual Functions and Dynamic Binding “Under the Hood”695
10.8 Virtual Destructors699
10.9 Case Study:Payroll System Using Polymorphism and Run-Time Type Information with dynamic_cast and typeid699
11 Templates718
11.1 Introduction719
11.2 Function Templates720
11.3 Overloading Function Templates723
11.4 Class Templates723
11.5 Class Templates and Nontype Parameters730
11.6 Templates and Inheritance731
11.7 Templates and Friends731
11.8 Templates and static Members732
12 C++ Stream Input/Output737
12.1 Introduction739
12.2 Streams739
12.2.1 Classic Streams vs.Standard Streams740
12.2.2 iostream Library Header Files740
12.2.3 Stream Input/Output Classes and Objects741
12.3 Stream Output743
12.3.1 Output of char * Variables743
12.3.2 Character Output using Member Function put744
12.4 Stream Input744
12.4.1 get and get line Member Functions745
12.4.2 istream Member Functions peek,putback and ignore748
12.4.3 Type-Safe I/O748
12.5 Unformatted I/O using read,write and gcount748
12.6 Introduction to Stream Manipulators749
12.6.1 Integral Stream Base:dec,oct,hex and setbase750
12.6.2 Floating-Point Precision (precision,setprecision)751
12.6.3 Field Width (width,setw)752
12.6.4 Programmer-Defined Manipulators754
12.7 Stream Format States and Stream Manipulators755
12.7.1 Trailing Zeros and Decimal Points (showpoint)756
12.7.2 Justification (left,right and internal)757
12.7.3 Padding (fill,setfill)759
12.7.4 Integral Stream Base (dec,oct,hex,showbase)760
12.7.5 Floating-Point Numbers; Scientific and Fixed Notation (scientific,fixed)761
12.7.6 Uppercase/Lowercase Control (uppercase)762
12.7.7 Specifying Boolean Format (boolalpha)763
12.7.8 Setting and Resetting the Format State via Member-Function flags764
12.8 Stream Error States766
12.9 Tying an Output Stream to an Input Stream768
13 Exception Handling779
13.1 Introduction780
13.2 Exception-Handling Overview781
13.3 Other Error-Handling Techniques783
13.4 Simple Exception-Handling Example:Divide by Zero784
13.5 Rethrowing an Exception788
13.6 Exception Specifications789
13.7 Processing Unexpected Exceptions790
13.8 Stack Unwinding790
13.9 Constructors,Destructors and Exception Handling792
13.10 Exceptions and Inheritance793
13.11 Processing new Failures793
13.12 Class auto_ptr and Dynamic Memory Allocation797
13.13 Standard Library Exception Hierarchy800
14 File Processing808
14.1 Introduction809
14.2 The Data Hierarchy809
14.3 Files and Streams811
14.4 Creating a Sequential-Access File812
14.5 Reading Data from a Sequential-Access File816
14.6 Updating Sequential-Access Files823
14.7 Random-Access Files824
14.8 Creating a Random-Access File824
14.9 Writing Data Randomly to a Random-Access File829
14.10 Reading Data Sequentially from a Random-Access File831
14.11 Example:A Transaction-Processing Program834
14.12 Input/Output of Objects841
15 Class string and String Stream Processing850
15.1 Introduction851
15.2 string Assignment and Concatenation852
15.3 Comparing strings855
15.4 Substrings857
15.5 Swapping strings858
15.6 string Characteristics859
15.7 Finding Strings and Characters in a string862
15.8 Replacing Characters in a string864
15.9 Inserting Characters into a string866
15.10 Conversion to C-Style char * Strings867
15.11 Iterators869
15.12 String Stream Processing870
16 Web Programming with CGI880
16.1 Introduction881
16.2 HTTP Request Types882
16.3 Multi-Tier Architecture882
16.4 Accessing Web Servers883
16.5 Apache HTTP Server884
16.6 Requesting XHTML Documents885
16.7 Introduction to CGI885
16.8 Simple HTTP Transaction886
16.9 Simple CGI Script888
16.10 Sending Input to a CGI Script895
16.11 Using XHTML Forms to Send Input897
16.12 Other Headers905
16.13 Case Study:An Interactive Web Page905
16.14 Cookies909
16.15 Server-Side Files915
16.16 Case Study:Shopping Cart921
16.17 Internet and Web Resources936
17 Data Structures942
17.1 Introduction943
17.2 Self-Referential Classes944
17.3 Dynamic Memory Allocation and Data Structures945
17.4 Linked Lists945
17.5 Stacks960
17.6 Queues965
17.7 Trees969
18 Bits,Characters,Strings and Structures1000
18.1 Introduction1001
18.2 Structure Definitions1001
18.3 Initializing Structures1003
18.4 Using Structures with Functions1004
18.5 typedef1004
18.6 Example:High-Performance Card-Shuffling and Dealing Simulation1005
18.7 Bitwise Operators1007
18.8 Bit Fields1017
18.9 Character-Handling Library1020
18.10 String-Conversion Functions1026
18.11 Search Functions of the String-Handling Library1031
18.12 Memory Functions of the String-Handling Library1036
19 Preprocessor1053
19.1 Introduction1054
19.2 The #include Preprocessor Directive1054
19.3 The #define Preprocessor Directive:Symbolic Constants1055
19.4 The #define Preprocessor Directive:Macros1056
19.5 Conditional Compilation1057
19.6 The #error and #pragma Preprocessor Directives1058
19.7 The # and ## Operators1059
19.8 Line Numbers1059
19.9 Predefined Symbolic Constants1060
19.10 Assertions1060
20 C Legacy Code Topics1065
20.1 Introduction1066
20.2 Redirecting Input/Output on UNIX and DOS Systems1066
20.3 Variable-Length Argument Lists1067
20.4 Using Command-Line Arguments1070
20.5 Notes on Compiling Multiple-Source-File Programs1071
20.6 Program Termination with exit and atexit1073
20.7 The volatile Type Qualifier1075
20.8 Suffixes for Integer and Floating-Point Constants1075
20.9 Signal Handling1075
20.10 Dynamic Memory Allocation with calloc and realloc1078
20.11 The Unconditional Branch:goto1079
20.12 Unions1080
20.13 Linkage Specifications1084
21 Standard Template Library (STL)1090
21.1 Introduction to the Standard Template Library (STL)1092
21.1.1 Introduction to Containers1094
21.1.2 Introduction to Iterators1098
21.1.3 Introduction to Algorithms1103
21.2 Sequence Containers1105
21.2.1 vector Sequence Container1105
21.2.2 l is t Sequence Container1113
21.2.3 deque Sequence Container1117
21.3 Associative Containers1119
21.3.1 multiset Associative Container1119
21.3.2 set Associative Container1122
21.3.3 mu l t imap Associative Container1124
21.3.4 map Associative Container1126
21.4 Container Adapters1128
21.4.1 stack Adapter1128
21.4.2 queue Adapter1130
21.4.3 priority_queue Adapter1132
21.5 Algorithms1133
21.5.1 fill,fill_n,generate and generate_n1134
21.5.2 equal,mismatch and lexicographical_compare1136
21.5.3 remove,remove_if,remove_copy and remove_copy_if1138
21.5.4 replace,replace_if,replace_copy and replace_copy_if1141
21.5.5 Mathematical Algorithms1144
21.5.6 Basic Searching and Sorting Algorithms1148
21.5.7 swap,iter_swap and swap_ranges1150
21.5.8 copy_backward,merge,unique and reverse1152
21.5.9 inplace_merge,unique_copy and reverse_copy1154
21.5.10 Set Operations1156
21.5.11 lower_bound,upper_bound and equal_range1160
21.5.12 Heapsort1162
21.5.13 min and max1165
21.5.14 Algorithms Not Covered in This Chapter1166
21.6 Class bitset1168
21.7 Function Objects1172
21.8 STL Internet and Web Resources1175
22 Other Topics1183
22.1 Introduction1184
22.2 const_cast Operator1184
22.3 reinterpret_cast Operator1185
22.4 namespaces1186
22.5 Operator Keywords1190
22.6 explicit Constructors1192
22.7 mutable Class Members1197
22.8 Pointers to Class Members (.* and ->*)1199
22.9 Multiple Inheritance1201
22.10 Multiple Inheritance and virtual Base Classes1205
22.11 Closing Remarks1210
A Operator Precedence Chart1214
B ASCII Character Set1216
C Number Systems1217
C.1 Introduction1218
C.2 Abbreviating Binary Numbers as Octal Numbers and Hexadecimal Numbers1221
C.3 Converting Octal Numbers and Hexadecimal Numbers to Binary Numbers1222
C.4 Converting from Binary,Octal or Hexadecimal to Decimal1222
C.5 Converting from Decimal to Binary,Octal or Hexadecimal1223
C.6 Negative Binary Numbers:Two’s Complement Notation1225
D C++ Internet and Web Resources1230
D.1 Resources1230
D.2 Tutorials1232
D.3 FAQs1233
D.4 Visual C++1233
D.5 Newsgroups1233
D.6 Compilers and Development Tools1234
D.7 Standard Template Library1234
E Introduction to XHTML1236
E.1 Introduction1237
E.2 Editing XHTML1237
E.3 First XHTML Example1238
E.4 Headers1240
E.5 Linking1242
E.6 Images1245
E.7 Special Characters and More Line Breaks1249
E.8 Unordered Lists1251
E.9 Nested and Ordered Lists1251
E.10 Basic XHTML Tables1252
E.11 Intermediate XHTML Tables and Formatting1257
E.12 Basic XHTML Forms1259
E.13 More Complex XHTML Forms1262
E.14 Internet and World Wide Web Resources1269
F XHTML Special Characters1274
Bibliography1275
Index1281